- Posted on
- • Linux
How to Append Text to File in Bash
- Author
-
-
- User
- edan
- Posts by this author
- Posts by this author
-
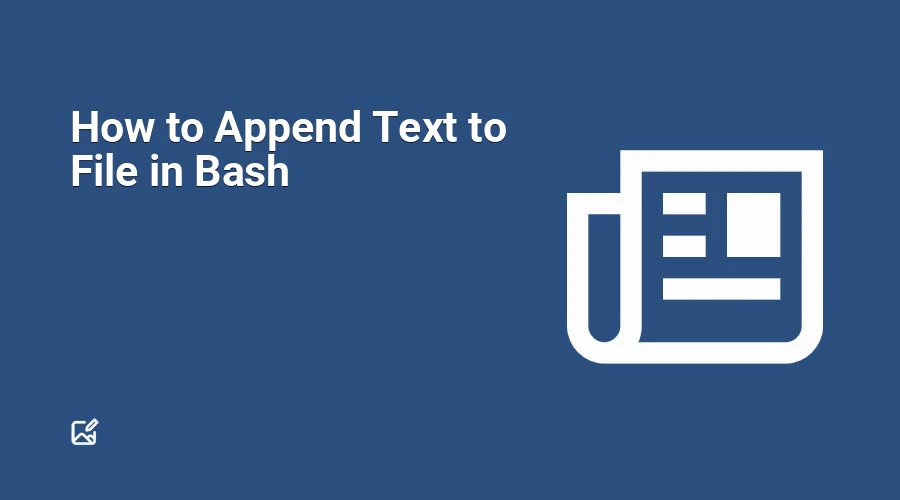
When working with Bash, there are various scenarios where appending text to files is necessary. From adding configuration details to logs or simply updating a text file, knowing how to append text efficiently using the command line can save time and effort. This article outlines several methods to append text to files, ensuring you can select the one that best suits your needs.
Using the Redirection Operator (>>
)
Bash provides a straightforward redirection operator (>>
) that allows you to append output from commands directly to a file. This method is intuitive and widely used for simple tasks.
To append a single line of text to a file, use the echo
command followed by the redirection operator and the file name:
echo "Adding a new line for logging purposes." >> my_log.txt
If you want to include special formatting, such as newline characters, the -e
option enables interpretation of backslash escapes:
echo -e "First entry:\nAdding a second line now." >> my_log.txt
For more advanced formatting options, the printf
command is ideal. It allows you to define the structure of your output with greater control:
printf "User %s logged in on %s\n" "$USER" "$(date)" >> my_log.txt
Using Here Documents (Heredoc)
Heredoc is a powerful Bash feature that allows for appending multiple lines of text at once. This method is useful when you want to include formatted blocks of text without repeating the redirection syntax.
For example, you can insert a brief summary of system status into a file as follows:
cat << EOF >> system_status.txt
Current directory: $PWD
User: $(whoami)
Timestamp: $(date)
EOF
You can also append the result of commands like date
directly into your logfile:
date +"Today is %A, the %d of %B, %Y" >> my_log.txt
Be cautious when using >
as this will overwrite the contents of a file instead of appending.
Appending Text with the tee
Command
The tee
command serves as another effective tool for appending text to files. Its versatility lies in its ability to read input and provide output both to the console and specified files simultaneously. By default, tee
overwrites existing content, but you can easily adjust this behavior using the -a
(append) option:
echo "Appending this text using tee." | tee -a my_log.txt
If you prefer to suppress the output on the terminal, you can direct it to /dev/null
:
echo "This will only append to the file." | tee -a my_log.txt > /dev/null
One of the key benefits of using tee
is its ability to work with files owned by other users, provided you have the necessary permissions. You can elevate your permissions using sudo
, enabling you to append to restricted files:
echo "Adding to a protected file." | sudo tee -a restricted_file.txt
To append the same content to multiple files simultaneously, simply list them:
echo "This text appears in multiple files." | tee -a file1.txt file2.txt file3.txt
Conclusion
Appending text to an existing file in Bash can be accomplished effectively using either the >>
redirection operator or the tee
command. The choice between the two depends on your specific requirements and workflow. The >>
operator is excellent for straightforward tasks, while tee
offers flexibility, especially when dealing with permissions or multiple files. With these techniques in hand, you can easily manage your text and log files in a Linux environment.