- Posted on
- • Uncategorized
Fixing PHP Curl HTTPS Certificate Authority Issues on Windows
- Author
-
-
- User
- edan
- Posts by this author
- Posts by this author
-
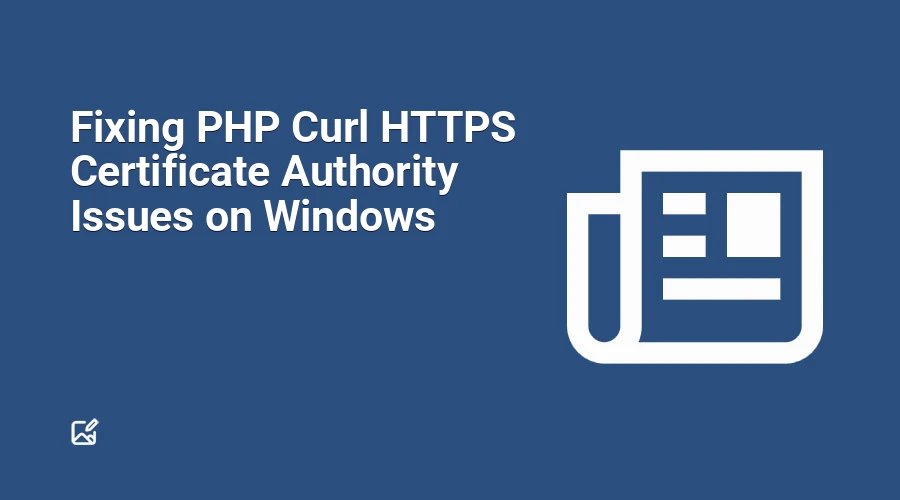
Making secure HTTPS requests in PHP using the Curl extension can sometimes result in certificate authority issues, particularly on Windows. This article explores effective solutions to address these issues related to the validation of TLS certificates in PHP Curl, ensuring secure connections without compromising security.
Troubleshooting HTTPS Requests
When attempting to make an HTTPS request using Curl, you might encounter an error indicating problems with the SSL certificate, such as:
$ch = curl_init('https://example.com');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if ($response === false) {
echo 'Curl Error: ' . curl_error($ch);
}
A typical error message could be: SSL certificate problem: self signed certificate in certificate chain
. This suggests that Curl cannot find or access the necessary root certificate file to validate the server's SSL certificate.
This challenge is more prevalent on Windows systems because, unlike macOS and Linux, there is no default file containing the list of trusted root certificates provided with PHP.
Utilizing Native Certificate Authorities
For those using Curl version 7.71 or later with PHP 8.2 or newer, leveraging the system's native certificate authorities is highly beneficial. By doing so, Curl can utilize the root certificates already present on the machine.
Implement this by configuring the Curl options as follows:
$ch = curl_init('https://example.com');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
if (defined('CURLSSLOPT_NATIVE_CA') && version_compare(curl_version()['version'], '7.71', '>=')) {
curl_setopt($ch, CURLOPT_SSL_OPTIONS, CURLSSLOPT_NATIVE_CA);
}
$response = curl_exec($ch);
This setup ensures that the request utilizes the native trust store effectively, enhancing security and reliability.
Managing a cacert.pem
File
When dealing with older PHP versions, or if your Curl version is below 7.71, the recommended method entails downloading and maintaining a cacert.pem
file that contains the necessary root certificates.
Follow these steps:
Download the
cacert.pem
: Obtain the latest version from the Curl project's official repository.Store the File: Place the downloaded file in an accessible location, like
C:/php/cacert.pem
.Update
php.ini
: Adjust the PHP configuration file to include the path to thecacert.pem
file:[curl] curl.cainfo = "C:/php/cacert.pem"
Restart the web server: If you're running a web server like Apache, restart it to apply changes.
The downside of this approach is that the cacert.pem
file should be periodically updated, as the certificate authorities are updated four to five times a year.
Alternatively, if modifying php.ini
is not an option, you can specify the path directly in your Curl request like this:
$ch = curl_init('https://example.com');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CAINFO, 'C:/php/cacert.pem');
$response = curl_exec($ch);
Avoiding Insecure Practices
While some online forums may suggest disabling SSL verification, this practice poses significant security risks. Ignoring certificate validation allows Curl to accept any TLS certificate, which could open the door to man-in-the-middle attacks.
Consider the following insecure approach, which should never be applied:
$ch = curl_init('https://example.com');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// DO NOT DISABLE VALIDATION
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
$response = curl_exec($ch);
Disabling these safety features is strongly discouraged and undermines the integrity of secure HTTPS communications.
Conclusion
Ensuring secure HTTPS requests in PHP on Windows requires proper configuration of the Curl extension to manage certificate authorities effectively. By leveraging native certificates or maintaining an updated cacert.pem
file, you can safeguard the integrity of your connections. It is critical to prioritize secure practices and avoid disabling SSL verification to maintain a robust security posture in your applications.