- Posted on
- • Uncategorized
How to Execute a Python Script
- Author
-
-
- User
- edan
- Posts by this author
- Posts by this author
-
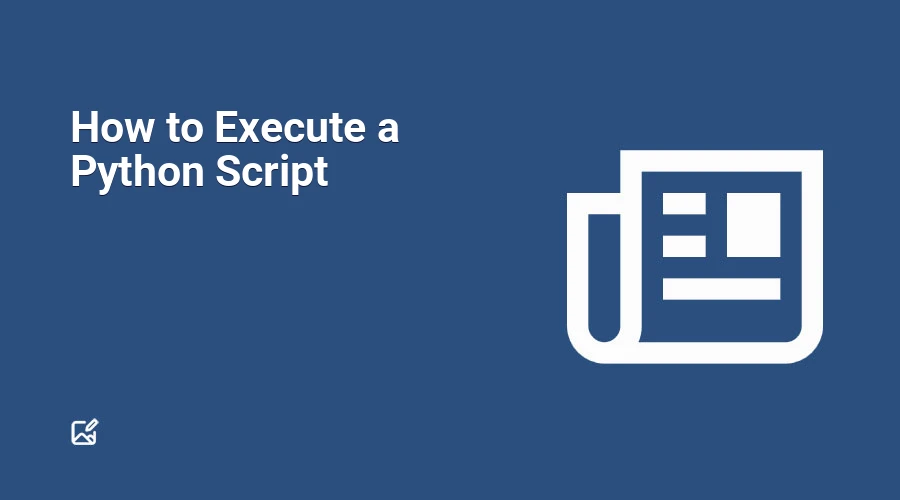
Python is widely recognized for its flexibility and readability, which makes it a favorite among developers and beginners alike. Running Python scripts is an integral part of programming with this versatile language. This guide will explore the various methods to execute Python scripts, including command-line methods, IDEs, and cloud-based platforms.
Initial Setup
Before proceeding, ensure that you have Python installed on your machine. To verify the installation, open your terminal or command prompt and run:
python --version
If Python is correctly installed, a version number will be displayed. Once confirmed, you’re all set to start running your scripts.
Writing Your First Script
Utilize an appropriate text editor or IDE that supports Python scripting, such as Atom, Visual Studio Code, or PyCharm. Create a file named greet.py
and enter the following code:
print("Welcome to Python scripting!")
Make sure to save the file in your preferred directory.
Executing a Python Script via Command Line
The command line is a powerful interface for executing scripts.
- Locate Your Script: Open your terminal and change directories to where your script is saved:
cd /path/to/your/script
- Run the Script: To execute your Python script from the command line, enter:
python greet.py
Upon pressing Enter, you should see the output:
Welcome to Python scripting!
Using Shebang for Easier Execution
Including a shebang line at the top of your script helps streamline execution on Unix-like systems, allowing you to run scripts without specifying the interpreter each time.
Add this line to the beginning of your greet.py
file:
#!/usr/bin/env python
After this, make your script executable by running the following command:
chmod +x greet.py
You can now execute the script directly with:
./greet.py
This should yield the same output as before.
Passing Arguments to Python Scripts
Python scripts can accept command-line arguments, adding versatility to your scripts. This enables users to customize their interactions with the script.
Here’s an enhanced example that takes user input:
import argparse
def main():
parser = argparse.ArgumentParser(description='A friendly greeting script.')
parser.add_argument('name', help='Name of the person to greet.')
args = parser.parse_args()
greet(args.name)
def greet(name):
print(f'Hello, {name}!')
if __name__ == '__main__':
main()
You can execute this script and input a name as follows:
python greet.py Alice
The output will be:
Hello, Alice!
Interactive Execution in the Python Shell
For quick tests and interactive code execution, you can use the Python interpreter. Start it by typing python
or python3
in your terminal. You will then see a prompt (>>>
) where you can immediately start typing Python commands.
Example interactions might include:
>>> 4 + 5
9
>>> x = "Hello, Python!"
>>> print(x)
Hello, Python!
Running Python Scripts in Integrated Development Environments (IDEs)
Many developers prefer using IDEs for their enriched development experience. Here’s how to run Python scripts in a couple of popular IDEs.
Using Visual Studio Code
- Ensure that you have Visual Studio Code installed.
- Open VS Code and navigate to the directory containing your Python script.
- Open the integrated terminal (View > Terminal) and type the following:
python greet.py
Press Enter, and the output will be displayed in the terminal.
Utilizing Jupyter Notebook
Jupyter Notebooks offer an interactive environment that is especially useful for data science tasks.
- Make sure you have Jupyter installed by running:
pip install notebook
- Start Jupyter by executing:
jupyter notebook
- In your browser, create a new Python notebook and enter your code in a cell. Press Shift + Enter to run the cell and see the output below.
Executing Scripts in Google Colab
Google Colab is an excellent platform for collaborative coding in the cloud. To run scripts here, follow these steps:
- Go to Google Colab in your web browser.
- Click on “File” followed by “New Notebook.”
- If your script requires access to files in Google Drive, insert the following to mount your drive:
from google.colab import drive
drive.mount('/content/drive')
- Upload your script, then execute it with:
!python /content/drive/MyDrive/path/to/greet.py
Remember to replace the path with the actual location of your script.
Conclusion
Mastering the execution of Python scripts is a vital skill in programming. From command-line execution to using advanced IDEs like Visual Studio Code and Jupyter Notebooks, there are numerous ways to run your scripts based on your preferences and needs. Each method enhances productivity in different contexts, and experimenting with these various approaches can be beneficial in developing your coding style.